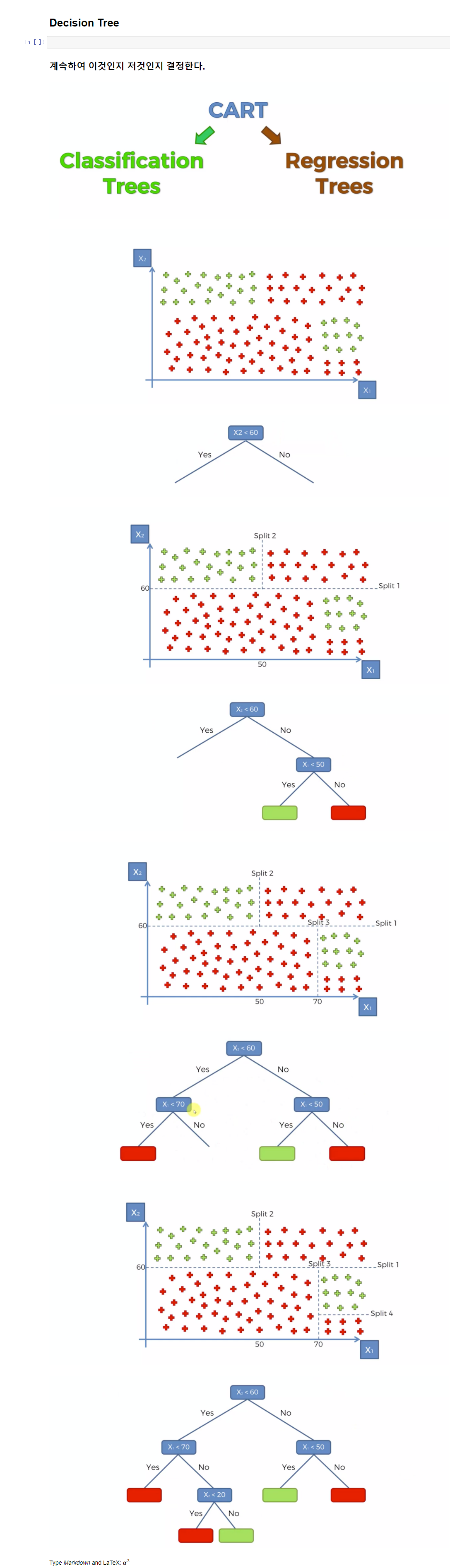
In [88]:
# Importing the libraries
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
In [89]:
df = pd.read_csv('../data/Social_Network_Ads.csv')
In [90]:
df
Out[90]:
User ID | Gender | Age | EstimatedSalary | Purchased | |
---|---|---|---|---|---|
0 | 15624510 | Male | 19 | 19000 | 0 |
1 | 15810944 | Male | 35 | 20000 | 0 |
2 | 15668575 | Female | 26 | 43000 | 0 |
3 | 15603246 | Female | 27 | 57000 | 0 |
4 | 15804002 | Male | 19 | 76000 | 0 |
... | ... | ... | ... | ... | ... |
395 | 15691863 | Female | 46 | 41000 | 1 |
396 | 15706071 | Male | 51 | 23000 | 1 |
397 | 15654296 | Female | 50 | 20000 | 1 |
398 | 15755018 | Male | 36 | 33000 | 0 |
399 | 15594041 | Female | 49 | 36000 | 1 |
400 rows × 5 columns
In [91]:
df.isna().sum()
Out[91]:
User ID 0 Gender 0 Age 0 EstimatedSalary 0 Purchased 0 dtype: int64
In [92]:
X= df.iloc[ :, [2,3] ]
In [93]:
y = df["Purchased"]
In [94]:
from sklearn.preprocessing import MinMaxScaler
In [95]:
scaler_x = MinMaxScaler()
In [96]:
X = scaler_x.fit_transform(X)
In [97]:
X
Out[97]:
array([[0.02380952, 0.02962963], [0.4047619 , 0.03703704], [0.19047619, 0.20740741], [0.21428571, 0.31111111], [0.02380952, 0.45185185], [0.21428571, 0.31851852], [0.21428571, 0.51111111], [0.33333333, 1. ], [0.16666667, 0.13333333], [0.4047619 , 0.37037037], [0.19047619, 0.48148148], [0.19047619, 0.27407407], [0.04761905, 0.52592593], [0.33333333, 0.02222222], [0. , 0.4962963 ], [0.26190476, 0.48148148], [0.69047619, 0.07407407], [0.64285714, 0.08148148], [0.66666667, 0.0962963 ], [0.71428571, 0.1037037 ], [0.64285714, 0.05185185], [0.69047619, 0.25185185], [0.71428571, 0.19259259], [0.64285714, 0.05185185], [0.66666667, 0.05925926], [0.69047619, 0.03703704], [0.73809524, 0.0962963 ], [0.69047619, 0.11111111], [0.26190476, 0.20740741], [0.30952381, 0.02222222], [0.30952381, 0.43703704], [0.21428571, 0.9037037 ], [0.07142857, 0.00740741], [0.23809524, 0.21481481], [0.21428571, 0.55555556], [0.4047619 , 0.08888889], [0.35714286, 0.0962963 ], [0.28571429, 0.25185185], [0.19047619, 0.42222222], [0.21428571, 0.11851852], [0.21428571, 0.01481481], [0.35714286, 0.26666667], [0.4047619 , 0.68888889], [0.28571429, 0. ], [0.23809524, 0.51111111], [0.11904762, 0.03703704], [0.16666667, 0.47407407], [0.21428571, 0.28888889], [0.28571429, 0.88888889], [0.30952381, 0.54814815], [0.14285714, 0.12592593], [0. , 0.21481481], [0.26190476, 0.5037037 ], [0.4047619 , 0.05925926], [0.21428571, 0.31851852], [0.14285714, 0.2962963 ], [0.11904762, 0.24444444], [0.23809524, 0.47407407], [0.0952381 , 0.02222222], [0.33333333, 0.75555556], [0.21428571, 0.03703704], [0.16666667, 0.53333333], [0.11904762, 0.37777778], [0.33333333, 0.77777778], [0.97619048, 0.5037037 ], [0.14285714, 0.31851852], [0.14285714, 0.02962963], [0.11904762, 0.4962963 ], [0.0952381 , 0.35555556], [0.30952381, 0.39259259], [0.16666667, 0.48148148], [0.14285714, 0.08888889], [0.04761905, 0.05925926], [0.35714286, 0.72592593], [0.33333333, 0.02222222], [0.38095238, 0.71851852], [0. , 0.27407407], [0.0952381 , 0.08888889], [0.23809524, 0.53333333], [0.19047619, 0.01481481], [0.28571429, 0.48148148], [0.5 , 0.2 ], [0.04761905, 0.25185185], [0.4047619 , 0.54074074], [0.28571429, 0.34814815], [0.30952381, 0.76296296], [0.14285714, 0.2962963 ], [0.23809524, 0.51851852], [0.19047619, 0.48888889], [0.4047619 , 0.25925926], [0.0952381 , 0.48888889], [0.28571429, 0.74814815], [0.19047619, 0. ], [0.26190476, 0.0962963 ], [0.26190476, 0.5037037 ], [0.4047619 , 0.21481481], [0.4047619 , 0.07407407], [0.23809524, 0.8 ], [0.4047619 , 0.42962963], [0.23809524, 0.16296296], [0.21428571, 0.54074074], [0.23809524, 0.32592593], [0.33333333, 0.52592593], [0.35714286, 0.99259259], [0.02380952, 0.04444444], [0.07142857, 0.42222222], [0.19047619, 0.14814815], [0.21428571, 0.54814815], [0.19047619, 0.52592593], [0.47619048, 0.48148148], [0.5 , 0.41481481], [0.45238095, 0.41481481], [0.47619048, 0.34074074], [0.45238095, 0.2962963 ], [0.57142857, 0.48148148], [0.52380952, 0.31111111], [0.4047619 , 0.44444444], [0.42857143, 0.27407407], [0.52380952, 0.32592593], [0.54761905, 0.32592593], [0.42857143, 0.44444444], [0.45238095, 0.42222222], [0.52380952, 0.44444444], [0.4047619 , 0.28148148], [0.54761905, 0.26666667], [0.5 , 0.34074074], [0.57142857, 0.37037037], [0.19047619, 0.12592593], [0.28571429, 0.01481481], [0.19047619, 0.51111111], [0.30952381, 0.31851852], [0.35714286, 0.11851852], [0.28571429, 0.53333333], [0.07142857, 0.39259259], [0.23809524, 0.2962963 ], [0.11904762, 0.35555556], [0.04761905, 0.4962963 ], [0.28571429, 0.68148148], [0.23809524, 0.32592593], [0.02380952, 0.07407407], [0.02380952, 0.51851852], [0. , 0.39259259], [0.4047619 , 0.32592593], [0.28571429, 0.54814815], [0.38095238, 0.07407407], [0.14285714, 0.54814815], [0.21428571, 0.6 ], [0.54761905, 0.11111111], [0.26190476, 0.34074074], [0.04761905, 0.43703704], [0.19047619, 0. ], [0.54761905, 0.22222222], [0.30952381, 0.45185185], [0.42857143, 0.25925926], [0.52380952, 0.23703704], [0.30952381, 0. ], [0.66666667, 0.32592593], [0.26190476, 0.44444444], [0.19047619, 0.11111111], [0.33333333, 0.88888889], [0.33333333, 0.62962963], [0.16666667, 0.55555556], [0.45238095, 0.13333333], [0.4047619 , 0.17037037], [0.35714286, 0.4 ], [0. , 0.52592593], [0.0952381 , 0.2962963 ], [0.4047619 , 0.41481481], [0.26190476, 0.98518519], [0.26190476, 0.23703704], [0.07142857, 0.54074074], [0.38095238, 0.74074074], [0.19047619, 0.76296296], [0.38095238, 0.20740741], [0.38095238, 0.42222222], [0.11904762, 0.0962963 ], [0.4047619 , 0.23703704], [0.16666667, 0.05185185], [0.14285714, 0.05925926], [0.30952381, 0.14074074], [0.19047619, 0.00740741], [0.30952381, 0.41481481], [0.33333333, 0.75555556], [0.35714286, 0.20740741], [0.35714286, 0.33333333], [0.30952381, 0.37777778], [0.04761905, 0.4962963 ], [0.35714286, 0.19259259], [0.4047619 , 0.42222222], [0.23809524, 0.12592593], [0.14285714, 0.51111111], [0.02380952, 0.08148148], [0.26190476, 0.20740741], [0.02380952, 0.40740741], [0.23809524, 0.54814815], [0.38095238, 0.20740741], [0.28571429, 0.47407407], [0.04761905, 0.15555556], [0.19047619, 0.48148148], [0.4047619 , 0.05185185], [0.4047619 , 0.17777778], [0.73809524, 0.43703704], [0.5 , 0.88148148], [0.54761905, 0.41481481], [0.95238095, 0.63703704], [0.69047619, 0.23703704], [0.88095238, 0.85185185], [0.80952381, 0.73333333], [0.52380952, 0.94074074], [0.66666667, 0.05185185], [0.71428571, 0.6 ], [0.80952381, 1. ], [0.97619048, 0.2 ], [0.4047619 , 0.31851852], [0.69047619, 0.20740741], [1. , 0.68888889], [0.73809524, 0.37037037], [0.52380952, 0.46666667], [0.66666667, 0.6 ], [0.97619048, 0.94814815], [0.54761905, 0.48148148], [0.4047619 , 0.56296296], [0.45238095, 0.95555556], [1. , 0.64444444], [0.4047619 , 0.33333333], [0.45238095, 0.28148148], [0.42857143, 0.82222222], [0.9047619 , 0.87407407], [0.52380952, 0.42222222], [0.57142857, 0.48148148], [0.4047619 , 0.97777778], [0.5 , 0.2 ], [0.52380952, 0.68148148], [0.73809524, 0.52592593], [0.47619048, 0.71851852], [0.66666667, 0.47407407], [0.52380952, 0.31111111], [0.45238095, 0.48148148], [0.66666667, 0.4962963 ], [0.83333333, 0.94814815], [0.57142857, 0.99259259], [0.47619048, 0.32592593], [0.76190476, 0.54074074], [0.9047619 , 0.65925926], [0.54761905, 0.42222222], [0.78571429, 0.97037037], [0.4047619 , 0.25925926], [0.92857143, 0.79259259], [0.54761905, 0.27407407], [0.4047619 , 0.60740741], [0.61904762, 0.17777778], [0.45238095, 0.27407407], [0.71428571, 0.88148148], [0.45238095, 0.97037037], [0.76190476, 0.21481481], [0.80952381, 0.55555556], [0.54761905, 0.42222222], [0.52380952, 0.31111111], [0.95238095, 0.59259259], [0.64285714, 0.85925926], [0.4047619 , 0.45925926], [0.42857143, 0.95555556], [0.88095238, 0.81481481], [0.4047619 , 0.42222222], [0.71428571, 0.55555556], [0.57142857, 0.68888889], [0.52380952, 0.44444444], [0.45238095, 0.43703704], [0.69047619, 0.95555556], [0.52380952, 0.34074074], [0.5952381 , 0.87407407], [0.97619048, 0.45185185], [1. , 0.2 ], [0.5 , 0.67407407], [0.92857143, 0.08148148], [0.92857143, 0.43703704], [0.47619048, 0.41481481], [0.73809524, 0.54074074], [0.80952381, 0.17037037], [0.76190476, 0.15555556], [0.97619048, 0.54074074], [0.4047619 , 0.34074074], [0.45238095, 0.40740741], [0.80952381, 0.04444444], [0.71428571, 0.93333333], [0.45238095, 0.57777778], [0.45238095, 0.34814815], [0.71428571, 0.91111111], [0.54761905, 0.47407407], [0.45238095, 0.46666667], [0.5 , 0.88148148], [0.73809524, 0.54814815], [0.88095238, 0.17777778], [0.45238095, 0.45925926], [0.4047619 , 0.31111111], [0.42857143, 0.35555556], [0.57142857, 0.42962963], [0.5952381 , 0.71851852], [0.64285714, 0.47407407], [0.66666667, 0.75555556], [0.95238095, 0.17037037], [0.71428571, 0.43703704], [0.45238095, 0.9037037 ], [0.45238095, 0.47407407], [0.52380952, 0.33333333], [0.57142857, 0.28888889], [0.78571429, 0.88148148], [0.69047619, 0.72592593], [0.42857143, 0.81481481], [0.47619048, 0.25925926], [0.57142857, 0.40740741], [0.5 , 0.6 ], [0.47619048, 0.25925926], [0.73809524, 0.93333333], [0.5 , 0.47407407], [0.5 , 0.44444444], [0.85714286, 0.65925926], [0.4047619 , 0.2962963 ], [0.64285714, 0.12592593], [0.42857143, 0.33333333], [0.80952381, 0.91111111], [0.83333333, 0.4962963 ], [0.54761905, 0.27407407], [0.71428571, 0.11111111], [0.71428571, 0.85925926], [0.54761905, 0.33333333], [0.54761905, 0.42222222], [0.57142857, 0.44444444], [0.42857143, 0.76296296], [0.69047619, 0.68148148], [0.47619048, 0.26666667], [0.71428571, 0.77037037], [0.57142857, 0.37037037], [0.52380952, 0.37037037], [0.92857143, 0.33333333], [0.42857143, 0.28888889], [0.95238095, 0.95555556], [0.4047619 , 0.47407407], [0.47619048, 0.2962963 ], [0.5 , 0.79259259], [0.83333333, 0.65925926], [0.4047619 , 0.44444444], [0.47619048, 0.37037037], [0.69047619, 0.26666667], [0.69047619, 0.66666667], [0.54761905, 0.35555556], [0.83333333, 0.42222222], [0.85714286, 0.68888889], [0.5 , 0.45925926], [0.47619048, 0.34074074], [0.47619048, 0.72592593], [0.45238095, 0.44444444], [0.57142857, 0.55555556], [0.45238095, 0.31111111], [0.42857143, 0.62222222], [1. , 0.14074074], [0.85714286, 0.40740741], [0.54761905, 0.42222222], [0.52380952, 0.41481481], [0.57142857, 0.28888889], [0.5952381 , 0.84444444], [0.83333333, 0.14074074], [0.69047619, 0.25925926], [0.57142857, 0.47407407], [0.57142857, 0.65925926], [0.97619048, 0.1037037 ], [0.95238095, 0.23703704], [0.66666667, 0.54074074], [0.47619048, 0.41481481], [0.85714286, 0.08148148], [1. , 0.22962963], [1. , 0.5037037 ], [0.5 , 0.42962963], [0.97619048, 0.85185185], [0.45238095, 0.48148148], [0.66666667, 0.12592593], [0.66666667, 0.43703704], [0.57142857, 0.28148148], [0.54761905, 0.53333333], [0.95238095, 0.05925926], [0.57142857, 0.36296296], [0.71428571, 0.13333333], [0.61904762, 0.91851852], [0.73809524, 0.0962963 ], [0.92857143, 0.13333333], [0.9047619 , 0.33333333], [0.73809524, 0.17777778], [0.5 , 0.41481481], [0.69047619, 0.14074074], [0.71428571, 0.14814815], [0.71428571, 0.13333333], [0.69047619, 0.05925926], [0.64285714, 0.22222222], [1. , 0.2 ], [0.5 , 0.32592593], [0.66666667, 0.19259259], [0.78571429, 0.05925926], [0.76190476, 0.03703704], [0.42857143, 0.13333333], [0.73809524, 0.15555556]])
In [98]:
from sklearn.model_selection import train_test_split
In [99]:
X_train, X_test, y_train, y_test = train_test_split(X,y, test_size=0.2, random_state=1)
In [100]:
# 모델링
In [101]:
from sklearn.tree import DecisionTreeClassifier
In [102]:
classifier = DecisionTreeClassifier(random_state=1)
In [103]:
classifier.fit(X_train, y_train)
Out[103]:
DecisionTreeClassifier(random_state=1)In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
DecisionTreeClassifier(random_state=1)
In [104]:
y_pred = classifier.predict(X_test)
In [105]:
y_pred
Out[105]:
array([0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0], dtype=int64)
In [106]:
y_test.values
Out[106]:
array([0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0], dtype=int64)
In [107]:
from sklearn.metrics import confusion_matrix, accuracy_score
In [108]:
cm = confusion_matrix(y_test, y_pred)
In [109]:
cm
Out[109]:
array([[42, 6], [ 9, 23]], dtype=int64)
In [110]:
(42+23) / cm.sum()
Out[110]:
0.8125
In [111]:
accuracy_score(y_test, y_pred)
Out[111]:
0.8125
In [ ]:
In [ ]:
In [ ]:
In [112]:
# Visualising the Test set results
from matplotlib.colors import ListedColormap
X_set, y_set = X_test, y_test
X1, X2 = np.meshgrid(np.arange(start = X_set[:, 0].min() - 1, stop = X_set[:, 0].max() + 1, step = 0.01),
np.arange(start = X_set[:, 1].min() - 1, stop = X_set[:, 1].max() + 1, step = 0.01))
plt.figure(figsize=[10,7])
plt.contourf(X1, X2, classifier.predict(np.array([X1.ravel(), X2.ravel()]).T).reshape(X1.shape),
alpha = 0.75, cmap = ListedColormap(('red', 'green')))
plt.xlim(X1.min(), X1.max())
plt.ylim(X2.min(), X2.max())
for i, j in enumerate(np.unique(y_set)):
plt.scatter(X_set[y_set == j, 0], X_set[y_set == j, 1],
c = ListedColormap(('red', 'green'))(i), label = j)
plt.title('Classifier (Test set)')
plt.xlabel('Age')
plt.ylabel('Estimated Salary')
plt.legend()
plt.show()
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points. *c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
In [ ]:
In [113]:
# Visualising the Training set results
from matplotlib.colors import ListedColormap
X_set, y_set = X_train, y_train
X1, X2 = np.meshgrid(np.arange(start = X_set[:, 0].min() - 1, stop = X_set[:, 0].max() + 1, step = 0.01),
np.arange(start = X_set[:, 1].min() - 1, stop = X_set[:, 1].max() + 1, step = 0.01))
plt.figure(figsize=[10,7])
plt.contourf(X1, X2, classifier.predict(np.array([X1.ravel(), X2.ravel()]).T).reshape(X1.shape),
alpha = 0.75, cmap = ListedColormap(('red', 'green')))
plt.xlim(X1.min(), X1.max())
plt.ylim(X2.min(), X2.max())
for i, j in enumerate(np.unique(y_set)):
plt.scatter(X_set[y_set == j, 0], X_set[y_set == j, 1],
c = ListedColormap(('red', 'green'))(i), label = j)
plt.title('Logistic Regression (Training set)')
plt.xlabel('Age')
plt.ylabel('Estimated Salary')
plt.legend()
plt.show()
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points. *c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
In [ ]:
In [114]:
# 디시전 트리의 성능을 개선한 모델이 있다.
# Random Forest
In [115]:
from sklearn.ensemble import RandomForestClassifier
In [116]:
classifier2 = RandomForestClassifier(n_estimators=100) # n_estimators => 디폴트는 100, Forest 에서의 나무갯수
In [117]:
classifier2.fit(X_train, y_train)
Out[117]:
RandomForestClassifier()In a Jupyter environment, please rerun this cell to show the HTML representation or trust the notebook.
On GitHub, the HTML representation is unable to render, please try loading this page with nbviewer.org.
RandomForestClassifier()
In [118]:
y_pred2 = classifier2.predict(X_test)
In [119]:
y_pred2
Out[119]:
array([0, 0, 1, 1, 1, 0, 0, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0], dtype=int64)
In [120]:
y_test.values
Out[120]:
array([0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0], dtype=int64)
In [121]:
confusion_matrix(y_test, y_pred2)
Out[121]:
array([[41, 7], [ 3, 29]], dtype=int64)
In [122]:
accuracy_score(y_test, y_pred2)
Out[122]:
0.875
In [123]:
df
Out[123]:
User ID | Gender | Age | EstimatedSalary | Purchased | |
---|---|---|---|---|---|
0 | 15624510 | Male | 19 | 19000 | 0 |
1 | 15810944 | Male | 35 | 20000 | 0 |
2 | 15668575 | Female | 26 | 43000 | 0 |
3 | 15603246 | Female | 27 | 57000 | 0 |
4 | 15804002 | Male | 19 | 76000 | 0 |
... | ... | ... | ... | ... | ... |
395 | 15691863 | Female | 46 | 41000 | 1 |
396 | 15706071 | Male | 51 | 23000 | 1 |
397 | 15654296 | Female | 50 | 20000 | 1 |
398 | 15755018 | Male | 36 | 33000 | 0 |
399 | 15594041 | Female | 49 | 36000 | 1 |
400 rows × 5 columns
In [ ]:
In [ ]:
# 나이가 38살이고, 연봉은 45000 달러다. 이 사람은 물건을 살것인가 말것인가?
In [124]:
new_data = np.array([38,45000])
In [125]:
new_data = new_data.reshape(1,2)
In [127]:
new_data = scaler_x.transform(new_data)
# scaler_X 는 이미 Minmax로 fit(학습) 한상태이기 때문에 transform 만 사용한다.
# warning이 뜨는 이유는 scale_x.fit_transform(X) 할때 X의 컬럼까지 스캐일햇기 때문
# 때문에, 워닝을 없애고 싶다면 scale_x.fit_transform(X.value) 를 넣는다.
C:\Users\5-10\Anaconda3\envs\YH\lib\site-packages\sklearn\base.py:450: UserWarning: X does not have valid feature names, but MinMaxScaler was fitted with feature names warnings.warn(
In [128]:
new_data
Out[128]:
array([[0.47619048, 0.22222222]])
In [129]:
classifier2.predict(new_data)
Out[129]:
array([0], dtype=int64)
In [130]:
import joblib
In [131]:
joblib.dump(classifier2, ' classifier2.pkl')
Out[131]:
[' classifier2.pkl']
In [132]:
joblib.dump(scaler_x, "scaler_x.pkl")
Out[132]:
['scaler_x.pkl']
In [ ]:
In [ ]:
##### 만들어진 인공지능을 서버에서 불러오는 단계를 표현. #####
In [ ]:
In [1]:
import joblib
In [5]:
classifier = joblib.load(" classifier2.pkl")
In [4]:
scaler_X = joblib.load("scaler_X.pkl")
In [6]:
import numpy as np
In [7]:
new_data = np.array([ 25, 70000])
In [9]:
new_data= new_data.reshape(1,2)
In [10]:
new_data = scaler_X.transform(new_data)
C:\Users\5-10\Anaconda3\envs\YH\lib\site-packages\sklearn\base.py:450: UserWarning: X does not have valid feature names, but MinMaxScaler was fitted with feature names warnings.warn(
In [11]:
new_data
Out[11]:
array([[0.16666667, 0.40740741]])
In [12]:
classifier.predict(new_data)
Out[12]:
array([0], dtype=int64)
In [ ]:
'DataScience > MachineLearning' 카테고리의 다른 글
Machine [unsupervised{Clustering(Hierarchical)}] (계층적, 병합적 군집)agglomerative (0) | 2022.12.05 |
---|---|
Machine [unsupervised{Clustering(K-means)}] (평할/분할 기반의 군집) (0) | 2022.12.05 |
Machine 유방암 데이터 분석 Grid search 활용, svm,corr(),heatmap (0) | 2022.12.02 |
Machine [supervised{Classification(K-Nearest Neighbor)}] (0) | 2022.12.02 |
Machine [supervised{Classification(Support Vector Machine)}] (0) | 2022.12.02 |